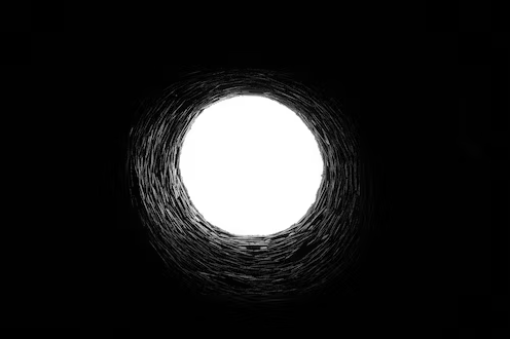
文字列には IsNullOrEmpty という、「null か、空だったら true」を返すメソッドがあります。
よくあるケースなので、結構便利です。
string message = null; string message2 = ""; string message3 = "abc"; Debug.Log(string.IsNullOrEmpty(message)); // true Debug.Log(string.IsNullOrEmpty(message2)); // true Debug.Log(string.IsNullOrEmpty(message3)); // false
まだ使ったことがない人は、これを使っておくとチェック漏れを防げるのでオススメ。
List でも同じことをしたい
List でも同じようなことをしたいのですが、残念ながら List.IsNullOr... はありません。
素直に書くならこのようなコードになりますが、
if (list == null || list.Count == 0)
{
// 空
}
毎回このように書くのも手間がかかりますし、== や || の記述をうっかり間違えたり、どちらか一方を忘れたりしそうですね。
そんな事故を防止するためにも、list に拡張メソッドを追加してみましょう。
Extensions_IEnumerable.cs
using System.Collections.Generic; using System.Linq; public static class Extensions_IEnumerable { public static bool IsNullOrEmpty<T>(this IEnumerable<T> self) { return self == null || self.Count() == 0; } }
使い方
List<int> list = null; List<int> list2 = new List<int>(); List<int> list3 = new List<int>() { 1, 2, 3 }; Debug.Log(list.IsNullOrEmpty()); // true Debug.Log(list2.IsNullOrEmpty()); // true Debug.Log(list3.IsNullOrEmpty()); // false
個人的には……
null や空だったら false の方が気分的に理解しやすいと常々思っている、でも、string.IsNullOrEmpty だからそれに合わせてる……。
でも、個人で使う分には逆転したメソッドもいいかもしれませんね。
using System.Collections.Generic; using System.Linq; public static class Extensions_IEnumerable { public static bool IsExists<T>(this IEnumerable<T> self) { return self != null && self.Count() > 0; } } public static class Extensions_String { public static bool IsExists(this string self) { return self != null && self.Length > 0; } }
複数人で開発する場合は、混乱を防ぐために出来るだけ基本に合わせましょう。